WordPress provides a flexible and powerful way for developers to extend and modify its functionality using hooks. One of the essential aspects of working with WordPress hooks is understanding how to query them effectively. This allows developers to locate, analyze, and manipulate hook functions efficiently.
Understanding WordPress Hooks 查询
WordPress hooks are mechanisms used to insert custom code into the core WordPress functions without modifying the core files. There are two primary types of hooks in WordPress:
- Action Hooks: These allow functions to execute at specific points in the WordPress runtime.
- Filter Hooks: These modify content before it is sent to the database or displayed on the screen.
Using these hooks, developers can add, modify, or remove functionality based on their requirements.
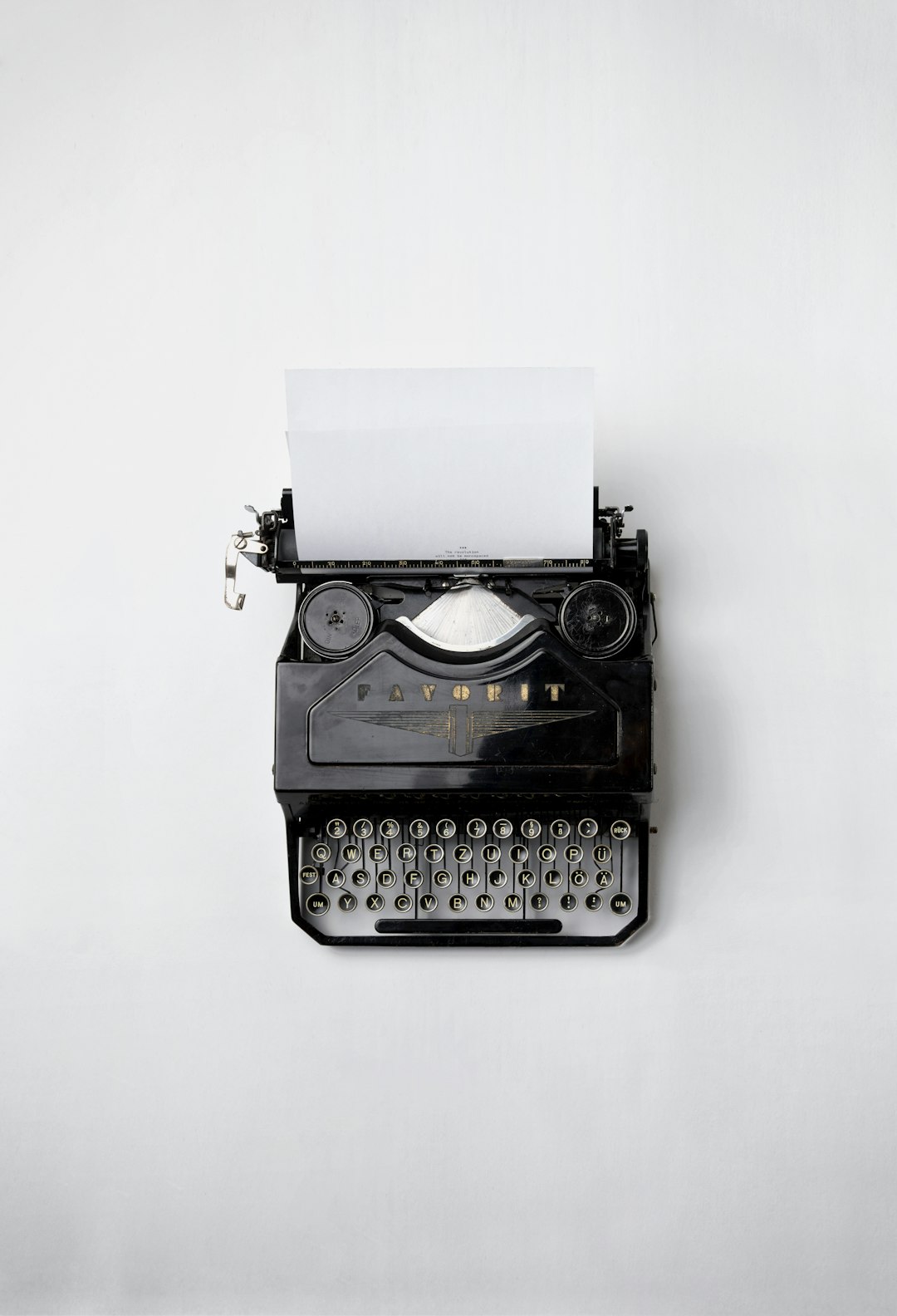
Querying WordPress Hook Functions
When working on a complex WordPress project, it is often necessary to query hook functions to see what functions are attached to a particular hook. This can help in debugging, modifying, or optimizing performance.
Using the wp_filter
Global Variable
WordPress stores all hooked functions in the global variable $wp_filter
. This allows developers to inspect and retrieve information about functions attached to specific hooks.
global $wp_filter;
print_r( $wp_filter['init'] );
The above code prints a list of all functions hooked to the init
action in the system.
Checking If a Function Is Hooked
To determine if a function is already hooked to a specific action or filter, WordPress provides the has_action()
and has_filter()
functions.
if ( has_action( 'wp_footer', 'custom_function' ) ) {
echo 'Function is hooked!';
}
Similarly, has_filter()
can check for filter hooks.
Retrieving Hooked Functions
To retrieve a list of all hooked functions for a specific action or filter, developers can use:
function get_hooked_functions( $hook_name ) {
global $wp_filter;
if ( isset( $wp_filter[ $hook_name ] ) ) {
return $wp_filter[ $hook_name ];
} else {
return 'No functions hooked to ' . $hook_name;
}
}
print_r( get_hooked_functions( 'wp_footer' ) );
This helps in getting a structured overview of the functions modifying or enhancing WordPress functionality.
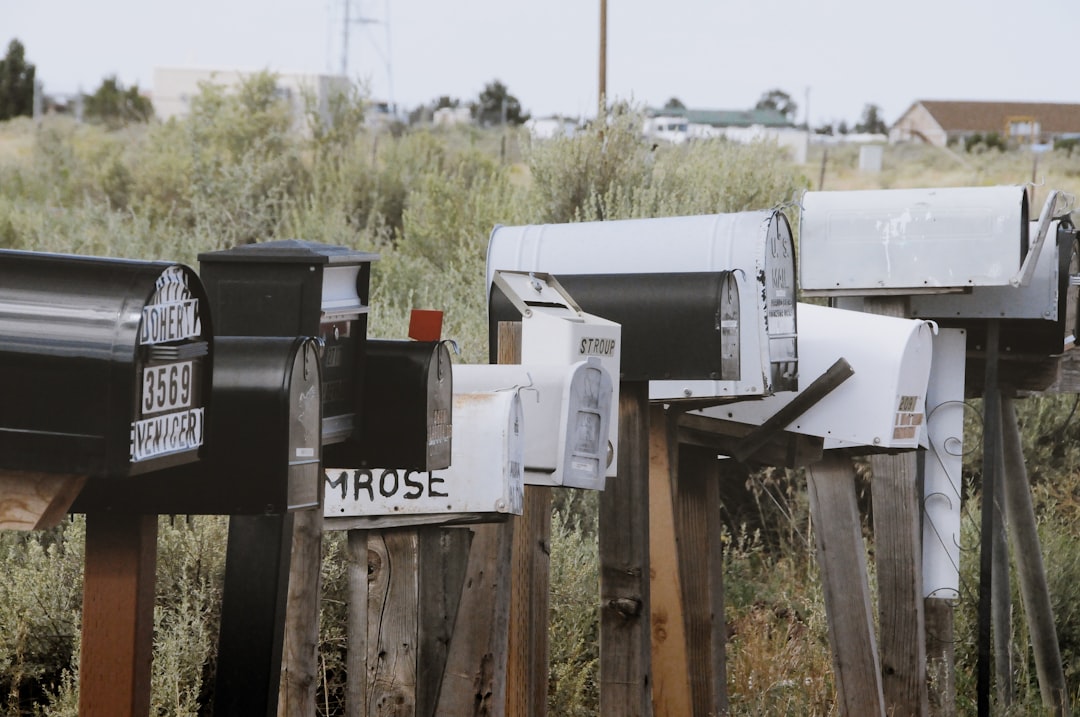
Debugging Hook Queries
For debugging purposes, developers can use WordPress debugging tools and plugins to inspect hook attachments. Some useful debugging techniques include:
- Using
print_r()
to inspect the$wp_filter
array. - Leveraging plugins like Query Monitor to visualize active hooks.
- Adding
do_action( 'hook_name' )
to manually trigger hooks and test behavior.
Conclusion
Querying WordPress hook functions is an essential skill for developers who wish to understand and modify WordPress behavior effectively. By leveraging global variables, built-in functions, and debugging tools, developers can easily analyze hooks and streamline their development process.
FAQs
What are WordPress hooks 查询?
WordPress hooks are predefined functions that allow developers to modify or extend the functionality of WordPress core without directly altering the core files.
How can I check if a function is hooked to an action?
You can use has_action( 'hook_name', 'function_name' )
to check if a function is attached to an action hook.
Where can I see all the hooks active on my site?
You can inspect the global $wp_filter
variable or use debugging plugins like Query Monitor to visualize hooks.
Can I remove a function from a hook?
Yes, you can use remove_action( 'hook_name', 'function_name' )
or remove_filter( 'filter_name', 'function_name' )
to detach functions.
What is the difference between actions and filters?
Actions allow the execution of functions at specific points, while filters modify data before it is displayed or processed further.